Thanks @FreddleSpl0it ,
If I omit defquota completely, or set it to 0, I get the error message “defquota empty”.
But when I set defquota to ANY positive number, I get “mailbox_defquota_exceeds_mailbox_maxquota”
The domain is set up correctly, and I can add mailboxes manually no problem (screenshot attached showing domain, with 5GB domain quota & 5GB max quota per mailbox)
Any help would be appreciated,
Here’s my python code:
#!/usr/bin/python3
import requests
import json
from pprint import pprint
url = "https://mailcow.example.com/api/v1/add/domain"
APIKey = "my-api-key"
headers = {
"Content-Type" : "application/json",
"X-API-Key" : APIKey
}
email_local_part = "test123"
domain = "ruonline-mailer.com.au"
password = "my-password"
data = {
"local_part": email_local_part,
"domain": domain,
"defquota": 1,
"quota": 1,
"password": password,
"password2": password,
"active": "1",
"tls_enforce_in": "1",
"tls_enforce_out": "1"
}
print("data:")
pprint(data)
r = requests.post(url, json=data, headers=headers)
print("API response:s")
pprint(r.json())
And here are some sample outputs showing the API response:
data:
{'active': '1',
'defquota': 0,
'domain': 'ruonline-mailer.com.au',
'local_part': 'test123',
'password': 'Mypassword12##',
'password2': 'Mypassword12##',
'quota': 0,
'tls_enforce_in': '1',
'tls_enforce_out': '1'}
API response:s
[{'log': ['mailbox',
'add',
'domain',
{'active': '1',
'defquota': 0,
'domain': 'ruonline-mailer.com.au',
'local_part': 'test123',
'password': '*',
'password2': '*',
'quota': 0,
'tls_enforce_in': '1',
'tls_enforce_out': '1'},
None],
'msg': 'defquota_empty',
'type': 'danger'}]
data:
{'active': '1',
'defquota': 1000,
'domain': 'ruonline-mailer.com.au',
'local_part': 'test123',
'password': 'Mypassword12##',
'password2': 'Mypassword12##',
'quota': 1000,
'tls_enforce_in': '1',
'tls_enforce_out': '1'}
API response:s
[{'log': ['mailbox',
'add',
'domain',
{'active': '1',
'defquota': 1000,
'domain': 'ruonline-mailer.com.au',
'local_part': 'test123',
'password': '*',
'password2': '*',
'quota': 1000,
'tls_enforce_in': '1',
'tls_enforce_out': '1'},
None],
'msg': 'mailbox_defquota_exceeds_mailbox_maxquota',
'type': 'danger'}]
And a screenshot showing my domain config:
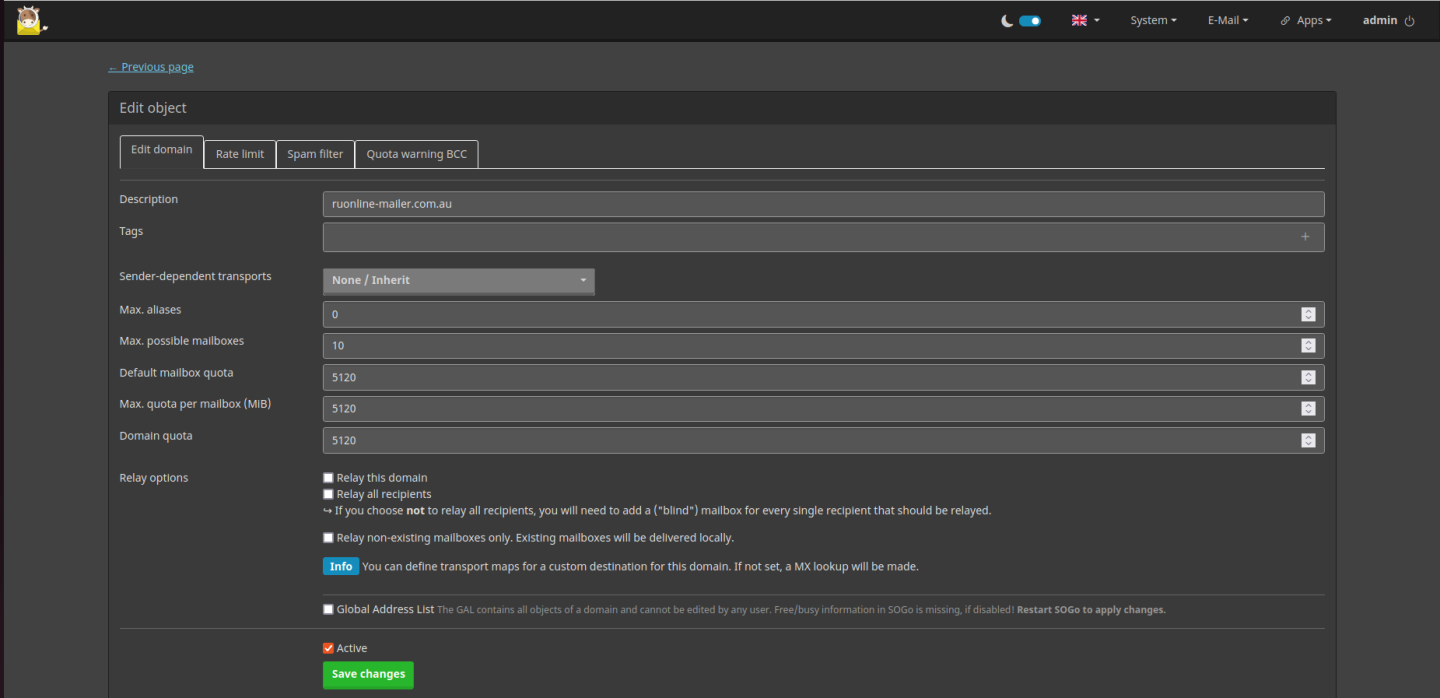